In some languages you can cast between strings and integers directly. This is not the case in Go, in Go you will need to parse your strings to convert them to integers.
This snippet will show you how to use the strconv
package in the standard library to parse strings. This package includes a variety of functions to help you convert between string and numeric types.
Using strconv.Atoi
The simplest way to convert a string to an integer in Go is by using the strconv.Atoi
function. โAtoiโ stands for โASCII to integerโ, based on the C function of the same name.
This function is an alias for strconv.ParseInt
which you will see later. strconv.Atoi
assumes a base of 10 and a bit size of 0.
In this example, strconv.Atoi
takes the string s
and attempts to convert it to an integer. If the conversion fails, it returns an error.
Using strconv.ParseInt
For more control over the conversion, you can use strconv.ParseInt
. This function allows you to specify the base and the bit size of the resulting integer. Hereโs an example:
In this example, strconv.ParseInt
parses string s
as a binary integer (base 2). The returned value will always be 64 bits, but only bits up to the provided bit size will be set.
strconv.ParseUint
instead.
Error handling
Donโt forget to handle your errors. Depending on the origin of the string, there is no guarantee that it will contain a valid integer.
In the above examples, we just print the errors to stdout. In real apps you probably want to log them, show an error to users or handle them in a different way.
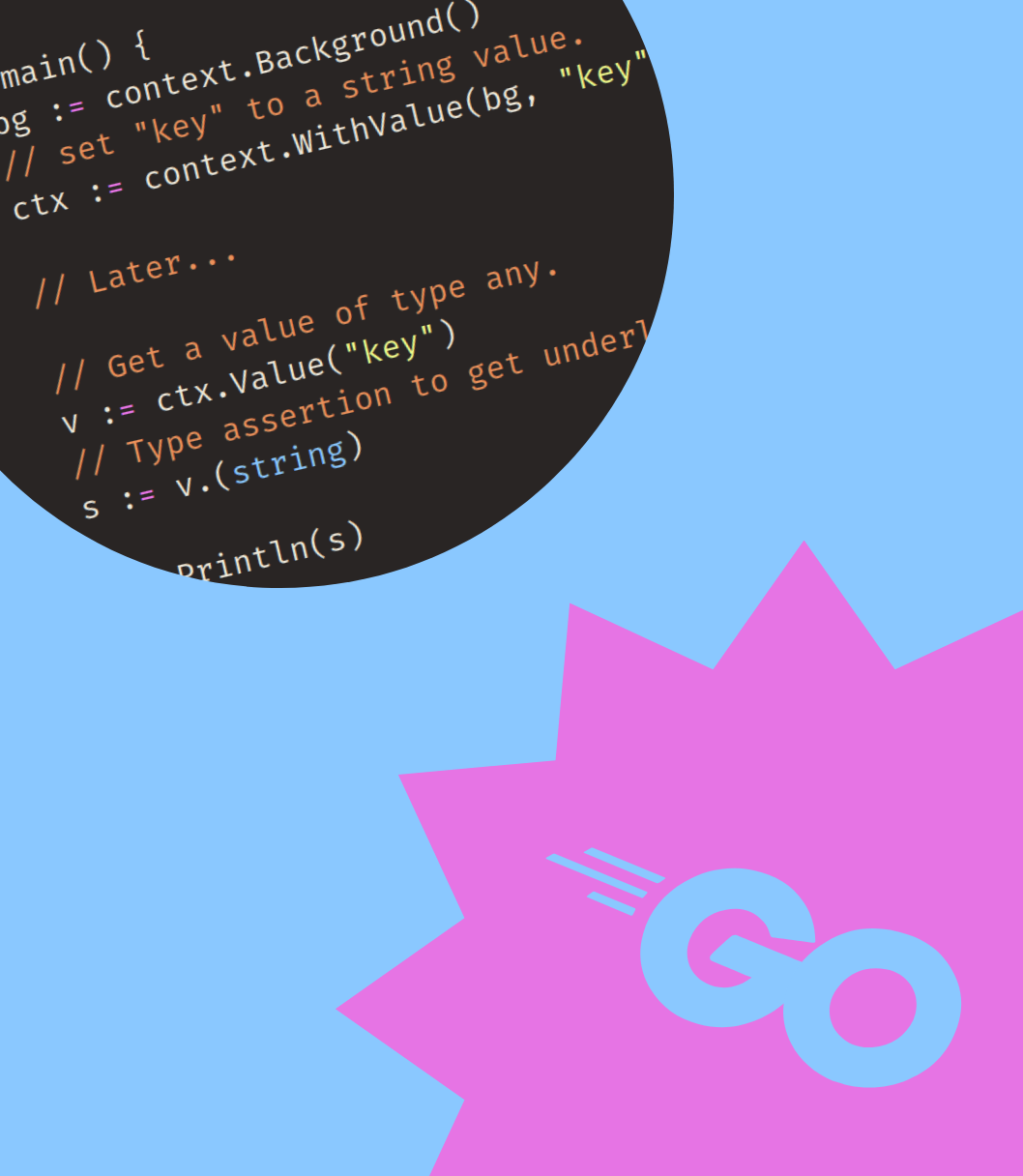
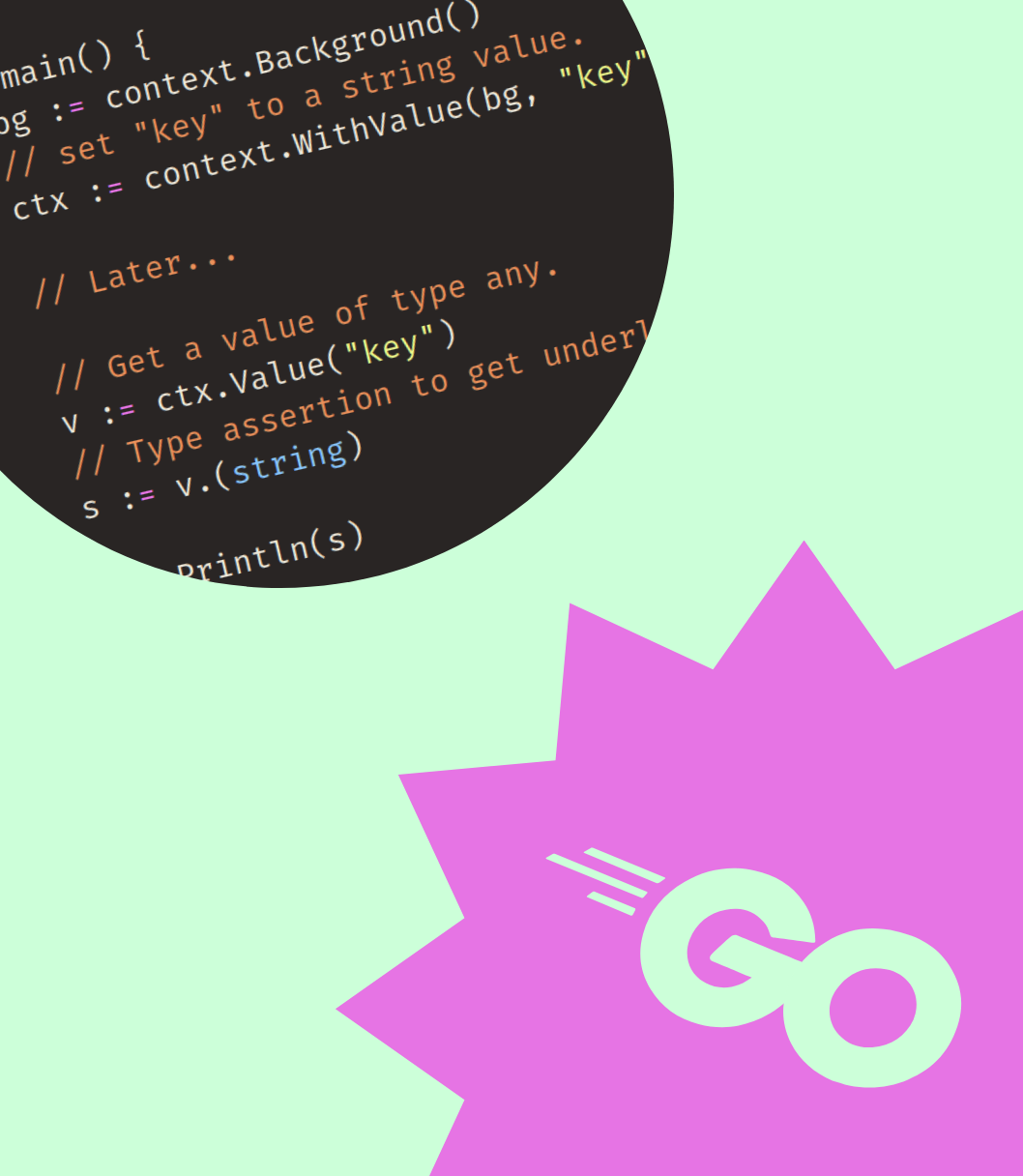
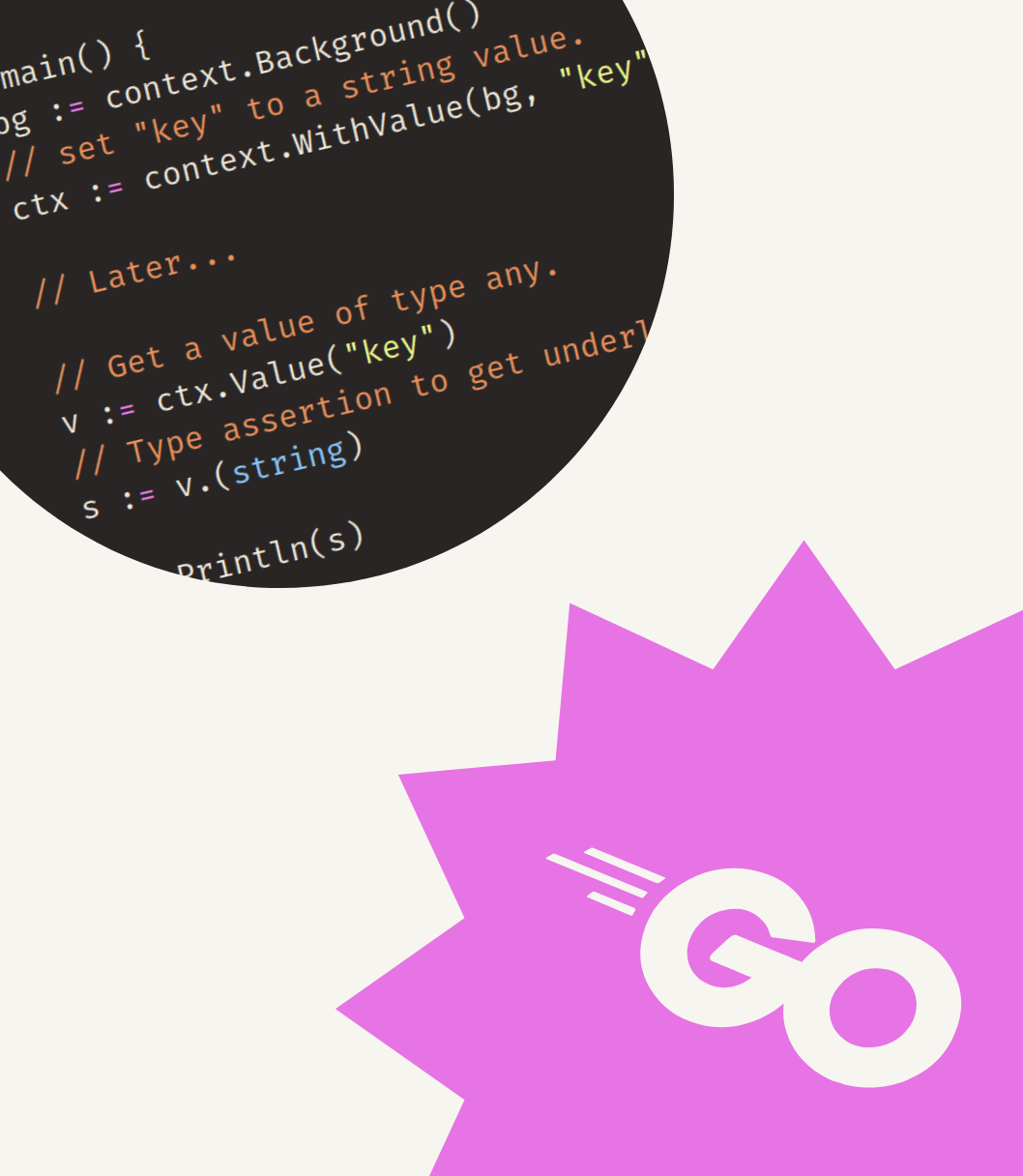
Get my free newsletter every second week
Used by 500+ developers to boost their Go skills.
"I'll share tips, interesting links and new content. You'll also get a brief guide to time for developers for free."
