Some languages have an in
operator that checks if a collection contains a certain value, Go does not.
In Go the same functionality is provided by a Contains
function.
Depending on your Go version you can use a different approach:
- In 1.21 and later, you can use the
Contains
function in theslices
package in the standard library. - For earlier versions, you can find the same function in the experimental
slices
package.
==
. Depending on your situation you may want to compare in a different way. The ContainsFunc
function allows you to specify a custom comparison function.
If you are using a Go version that does not support generics, you can always implement your own SliceContains
function. See the example below:
package main
import (
"fmt"
"slices"
)
func main() {
s := []string{"🌱", "🪡", "🌱"}
x := "🪡"
y := "🙈"
fmt.Println("slices.Contains:")
fmt.Printf("s contains \"%s\"? %v\n", x, slices.Contains(s, x))
fmt.Printf("s contains \"%s\"? %v\n", y, slices.Contains(s, y))
fmt.Println("---")
fmt.Println("SliceContains:")
fmt.Printf("s contains \"%s\"? %v\n", x, SliceContains(s, x))
fmt.Printf("s contains \"%s\"? %v\n", y, SliceContains(s, y))
}
// SliceContains returns true if s contains v.
func SliceContains(s []string, v string) bool {
for _, vs := range s {
if vs == v {
return true
}
}
return false
}
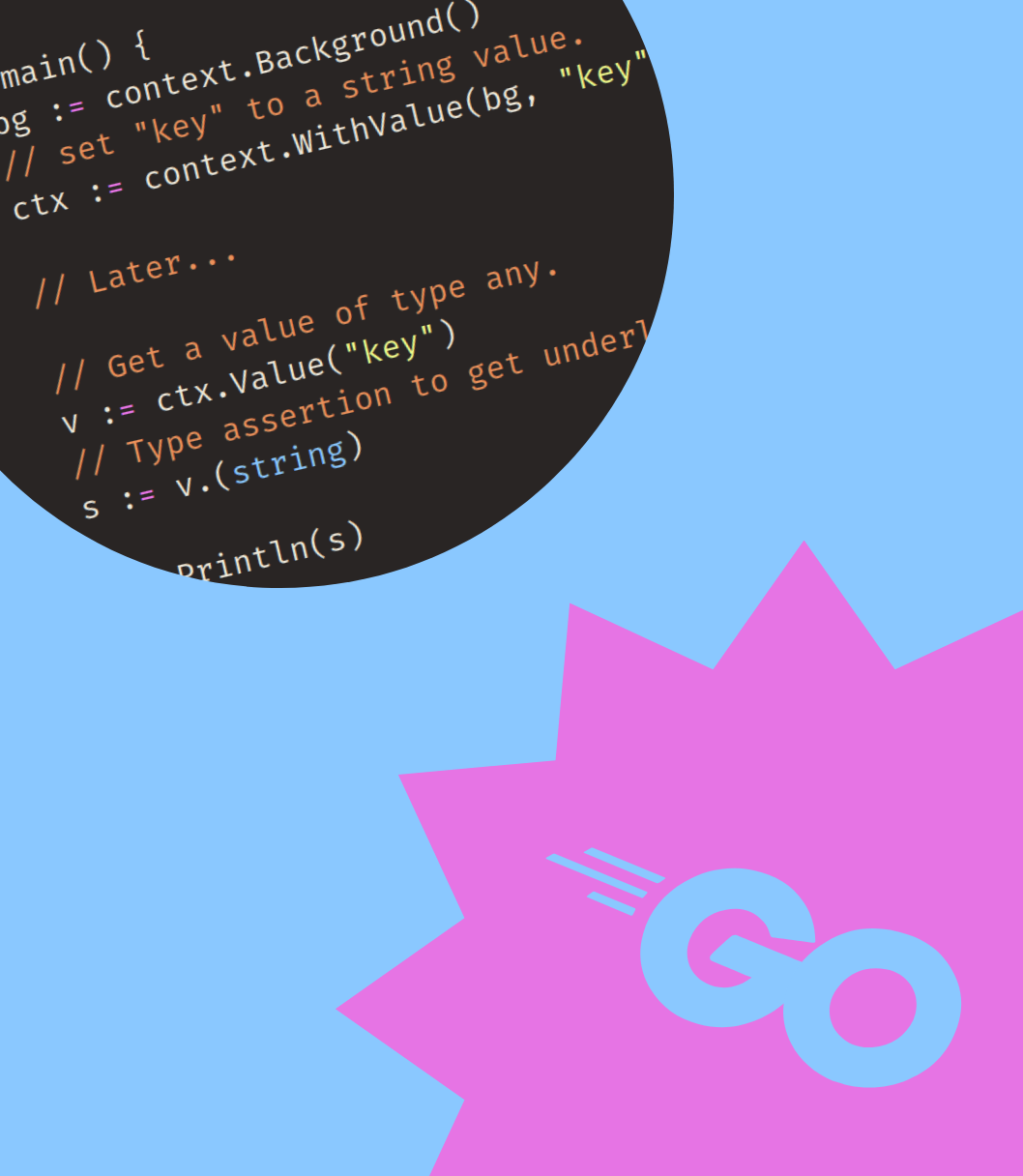
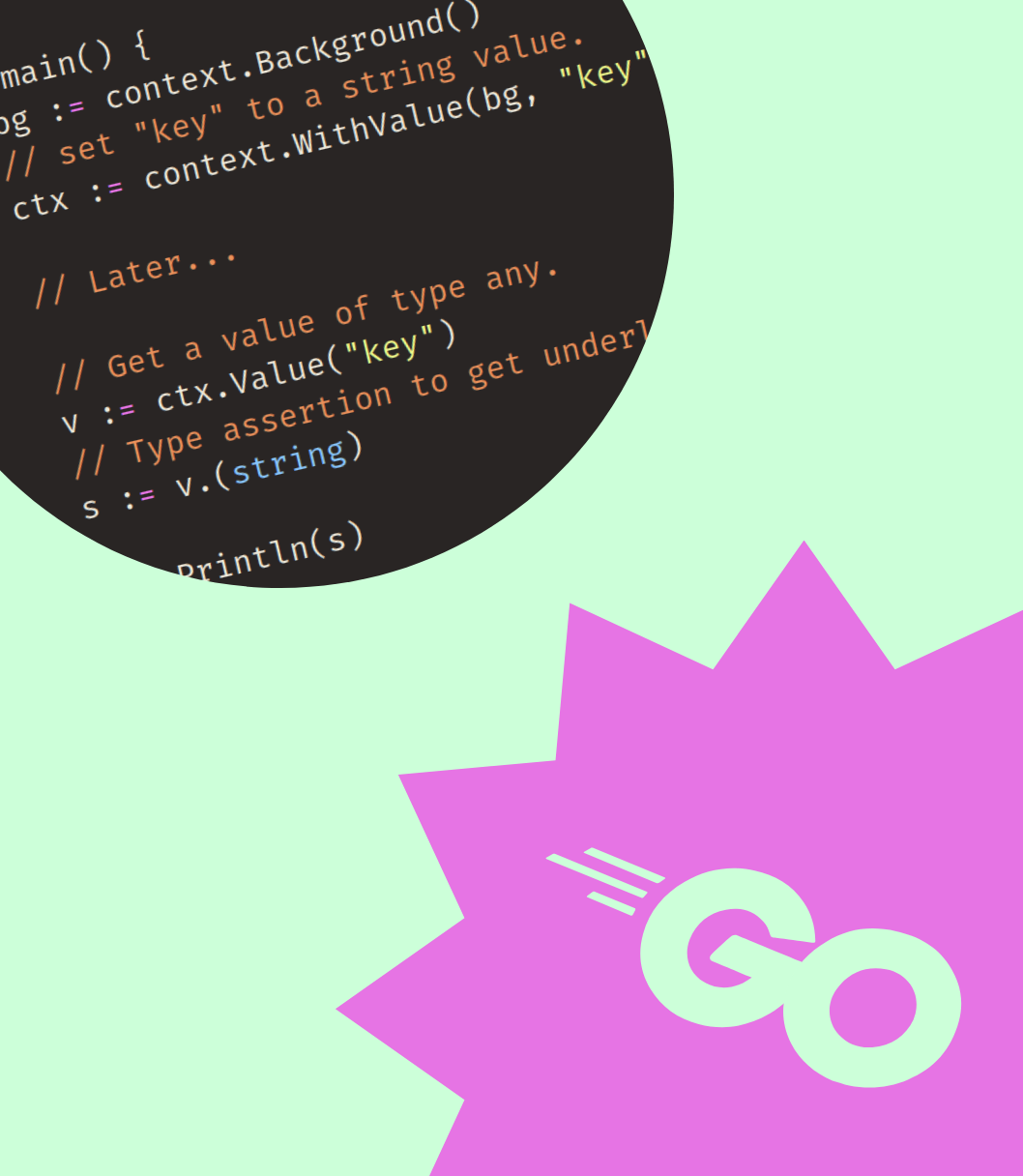
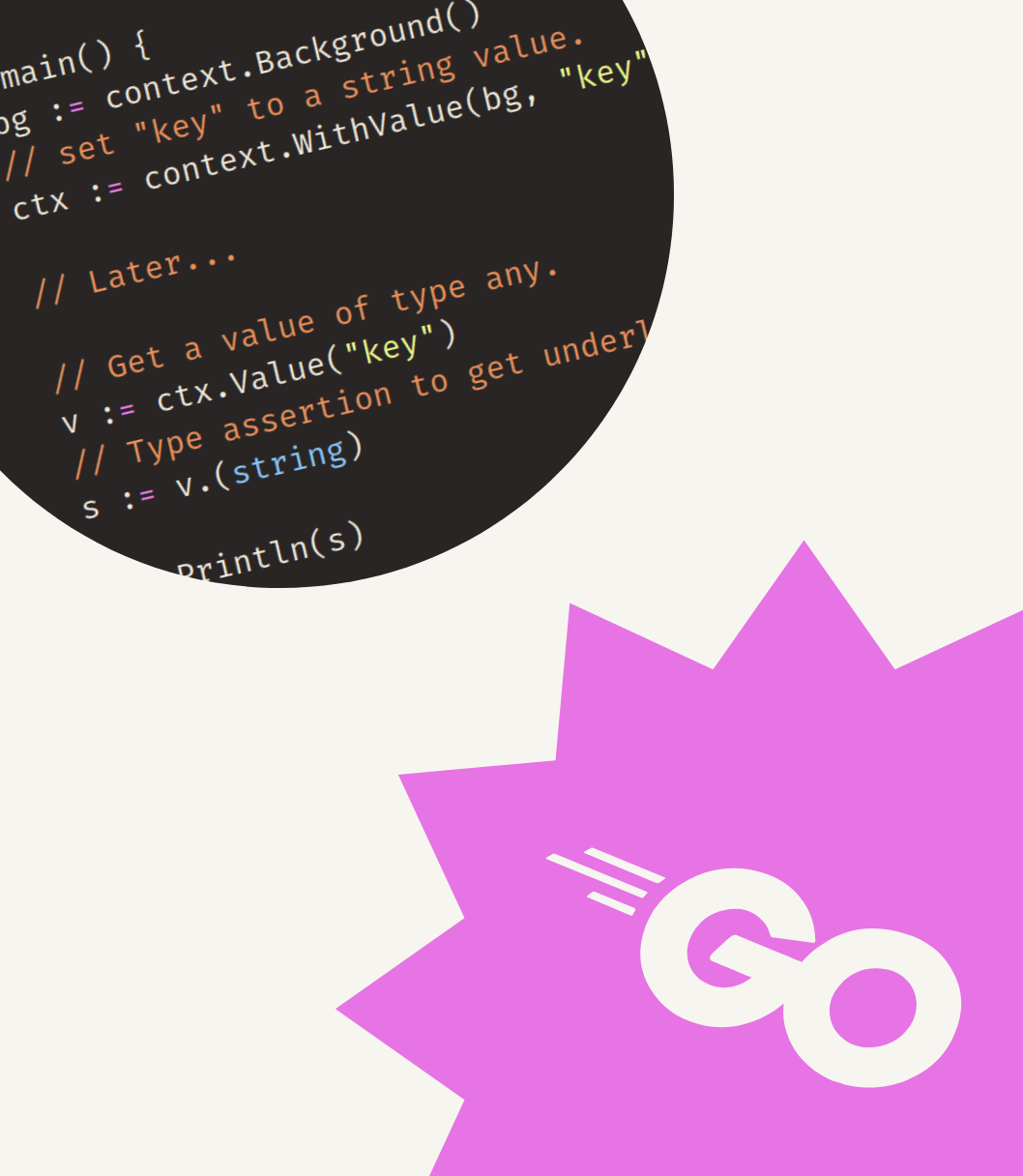
Get my free newsletter periodically*
Used by 500+ developers to boost their Go skills.
*working on a big project as of 2025, will get back to posting on the regular schedule once time allows.
