In some applications it can be useful to accept only times as input, not times with dates.
For example, in scheduling or time-tracking scenarios there can be a need for “time of the day” over “full timestamp” inputs.
There are some things you need to consider when parsing times in Go:
- What format are you expecting? 24-hour or 12-hour?
- What precision do you need? Hours, minutes, seconds?
- Be aware of default values for other
time.Time
elements.
24 Hour Format
In the 24-hour format the hours of the day are marked from midnight to midnight. The first hour begins at 00:00
and the days last hour at 23:00
(formatted as hours:minutes
).
In Go you can use the reference layout "15:04"
to parse times like this.
If you want to include seconds as well, you can use the existing constant time.TimeOnly
:
// ...
TimeOnly = "15:04:05"
// ...
12 Hour (AM/PM) Format
In the 12-hour format the day is split into two 12-hour periods:
- a.m. from Latin “ante meridiem”, meaning “before midday”.
- p.m. from Latin “post meridium”, meaning “after midday”.
The first hour of the day starts at 12:00AM
(“12 midnight”) and the last hour begins at 11:00PM
.
When you only need minute-precision we can use the existing time.Kitchen
constant:
// ...
Kitchen = "3:04PM"
// ...
If you need second-precision you can use the following reference layout: "3:04:05PM"
.
Other elements default to zero
Be aware that all elements of a time.Time
that are not part of the time (years, days, location) will correspond to their default values.
- Year and day will default to
1st of January 0000
. - Location will default to the
UTC
Location.
If your app needs to handle these times in a different context, be sure to set the appropriate date and location.
package main
import (
"fmt"
"log"
"time"
)
func parseAndPrint(layout, in string) {
t, err := time.Parse(layout, in)
if err != nil {
log.Fatalf("failed to parse %s: %v", in, err)
}
fmt.Println(t)
}
func main() {
// 24-hour: hours and minutes.
parseAndPrint("15:04", "13:37")
// 24-hour: hours, minutes and seconds.
parseAndPrint(time.TimeOnly, "13:37:42")
// 12-hour: hours and minutes.
parseAndPrint(time.Kitchen, "1:37PM")
// 12-hour: hours, minutes and seconds.
parseAndPrint("3:04:05PM", "1:37:42PM")
}
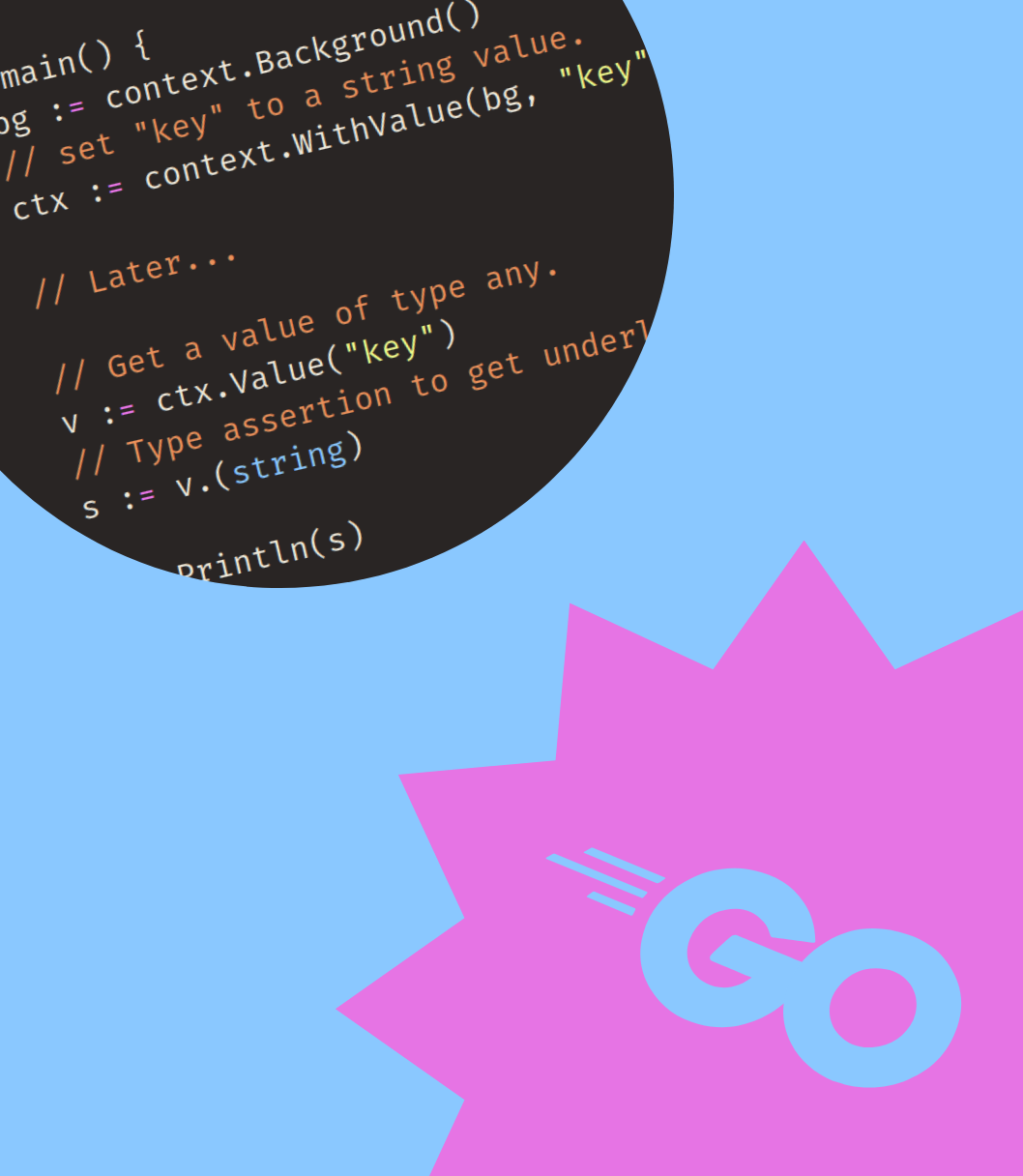
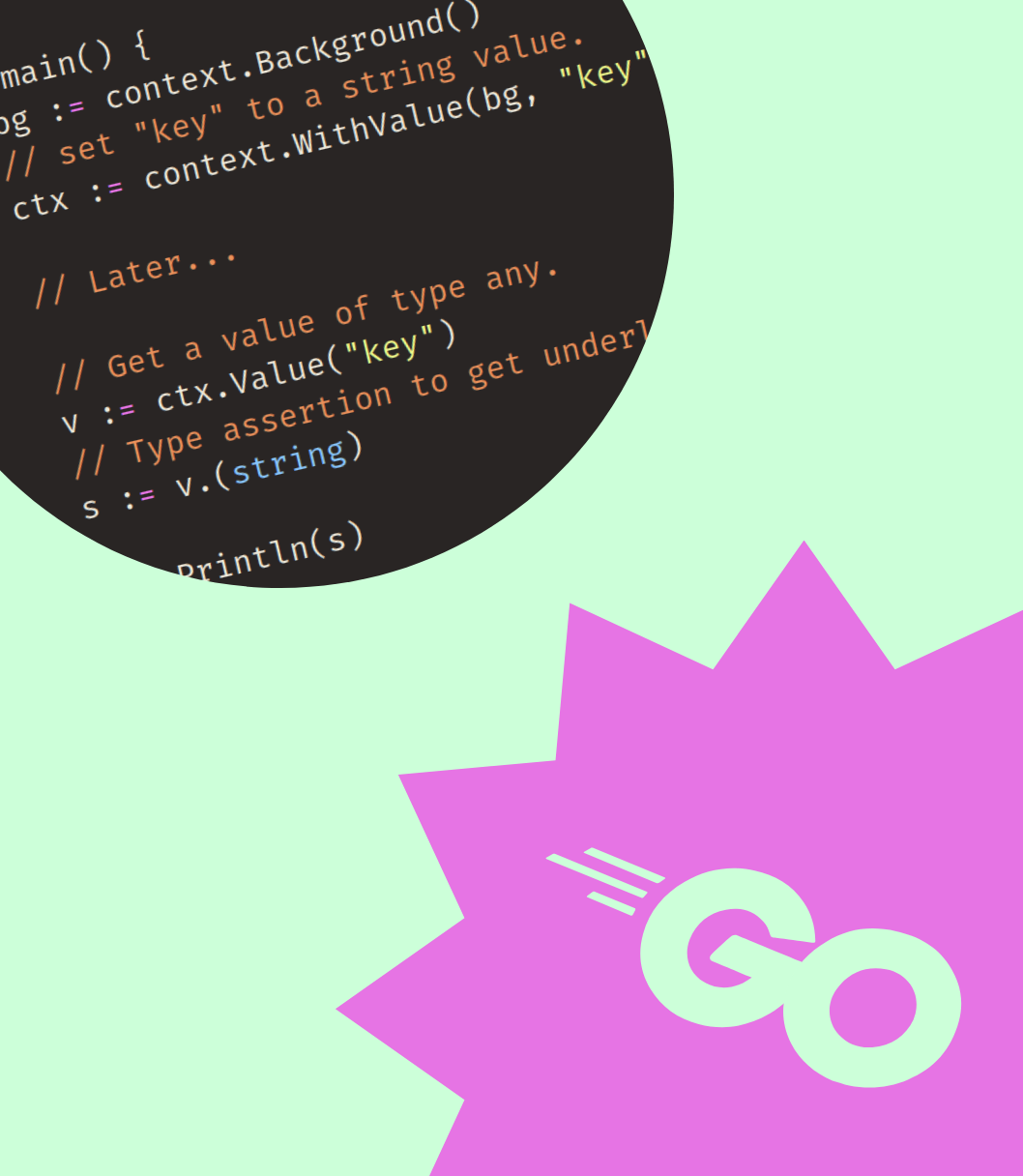
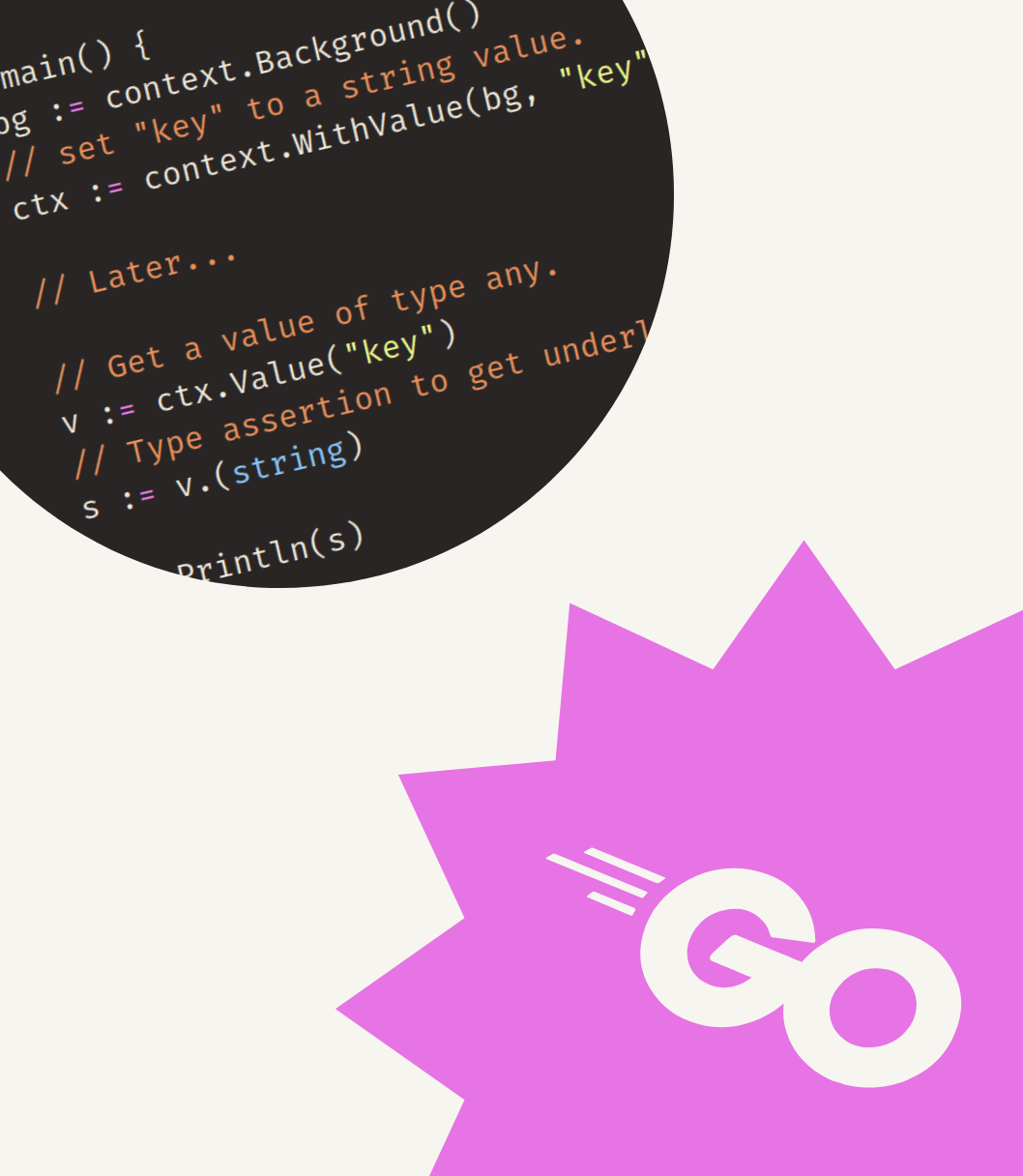
Get my free newsletter every second week
Used by 500+ developers to boost their Go skills.
"I'll share tips, interesting links and new content. You'll also get a brief guide to time for developers for free."
