In Go, you can’t directly cast an int to a string as you might in more loosely typed languages. Instead, you’ll need to format your integer as a string using one of several options.
This snippet will show you how to use the strconv
and fmt
packages to format integers as strings.
Using strconv.Itoa
The simplest way to convert an integer to a string is by using the strconv.Itoa
function. This function will take your integer and format it as a base 10 integer.
“Itoa” stands for “Integer to ASCII”, based on a common C function of the same name.
See the example below:
package main
import (
"fmt"
"strconv"
)
func main() {
i := 12345
s := strconv.Itoa(i)
fmt.Println("string:", s)
}
In this example the i
integer is converted to string s
using strconv.Itoa
and then printed to stdout.
Using strconv.FormatInt
If you want to format your integer in a different base, strconv.FormatInt
is the right choice.
For example, you can format your integer in binary (base 2) as follows:
package main
import (
"fmt"
"strconv"
)
func main() {
i := int64(5) // strconv.FormatInt requires int64.
s := strconv.FormatInt(i, 2)
fmt.Println("string:", s)
}
Bases up to base 36 are supported, if an invalid base is provided the function will panic.
strconv.FormatUint
instead.
Using the fmt package
So far we’ve only converted the integer to a string without including more text. But what if you want to include more?
You should take a look at the fmt
package.
For example, the fmt.Sprintf
function allows you to specify a layout string with a placeholder for the integer.
package main
import (
"fmt"
)
func main() {
i := 12345
s := fmt.Sprintf("%d is the number", i)
fmt.Println(s)
}
In this example, %d
is the placeholder for a base 10 integer.
Other integer placeholders include:
placeholder | format |
---|---|
%b |
base 2 |
%c |
the character represented by the corresponding Unicode code point |
%d |
base 10 |
%o |
base 8 |
%O |
base 8 with 0o prefix |
%q |
a single-quoted character literal safely escaped with Go syntax. |
%x |
base 16, with lower-case letters for a-f |
%X |
base 16, with upper-case letters for A-F |
%U |
Unicode format: U+1234 ; same as "U+%04X" |
In the fmt
package documentation you can also find several flags that enable extra options for these placeholders.
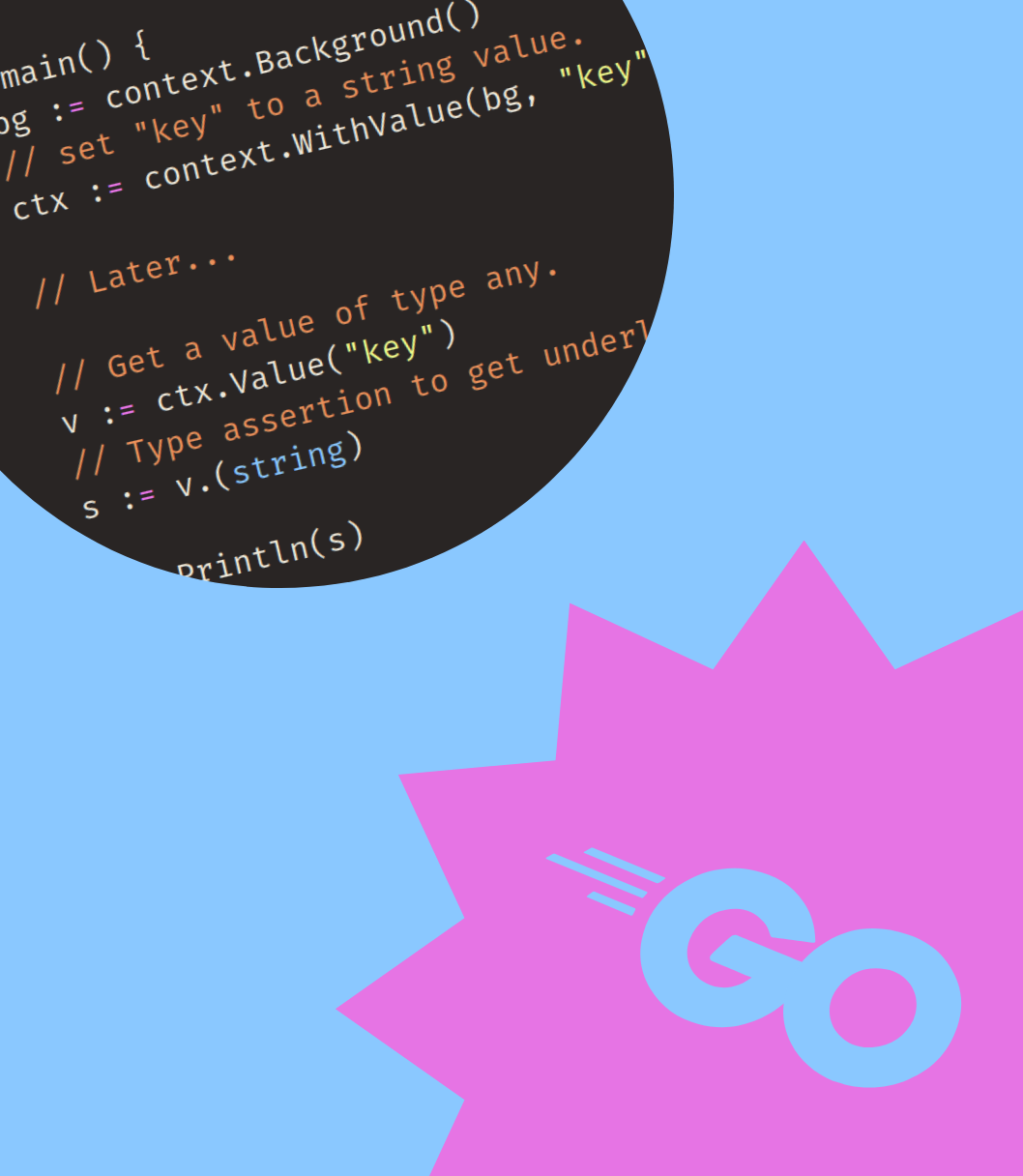
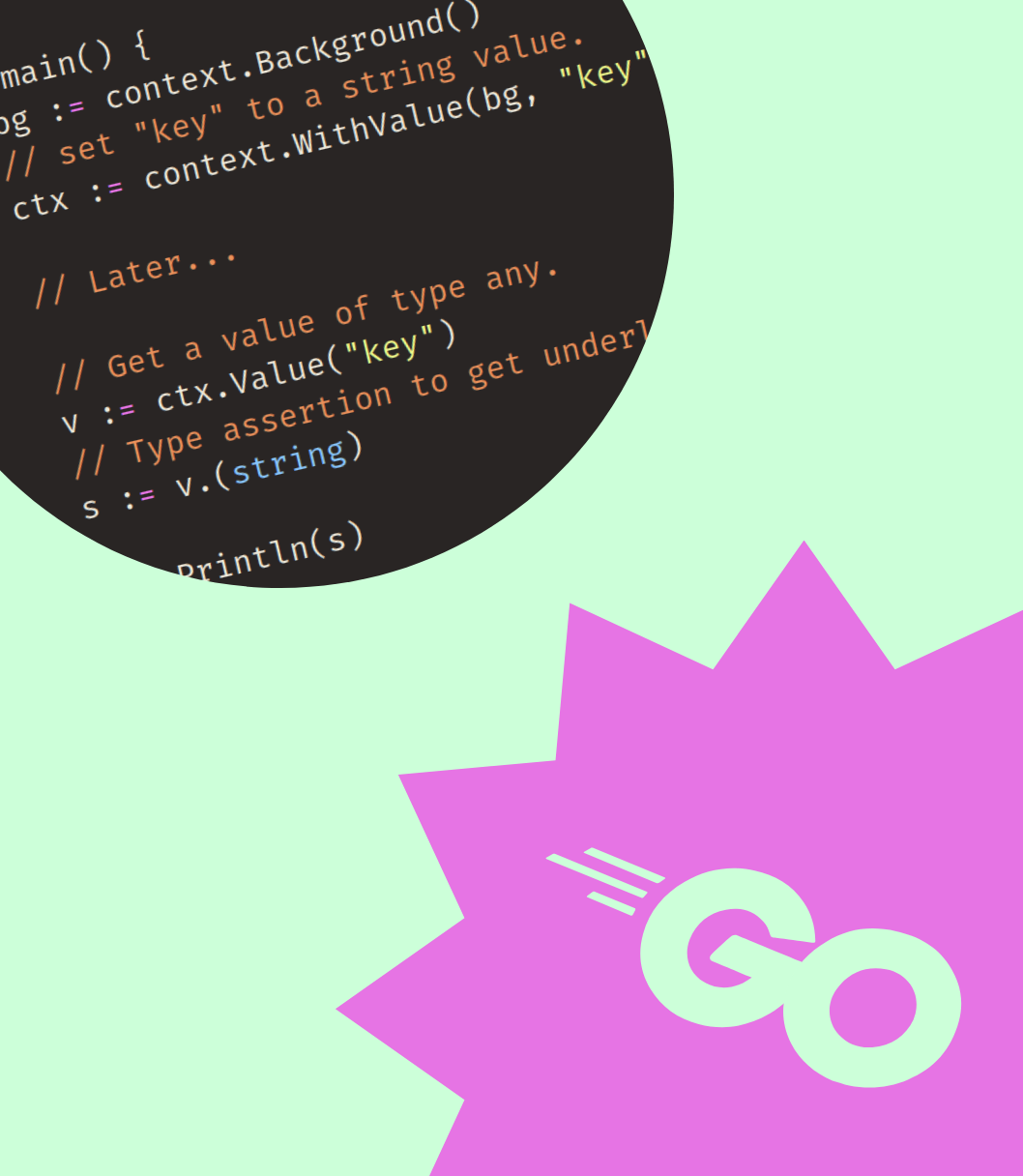
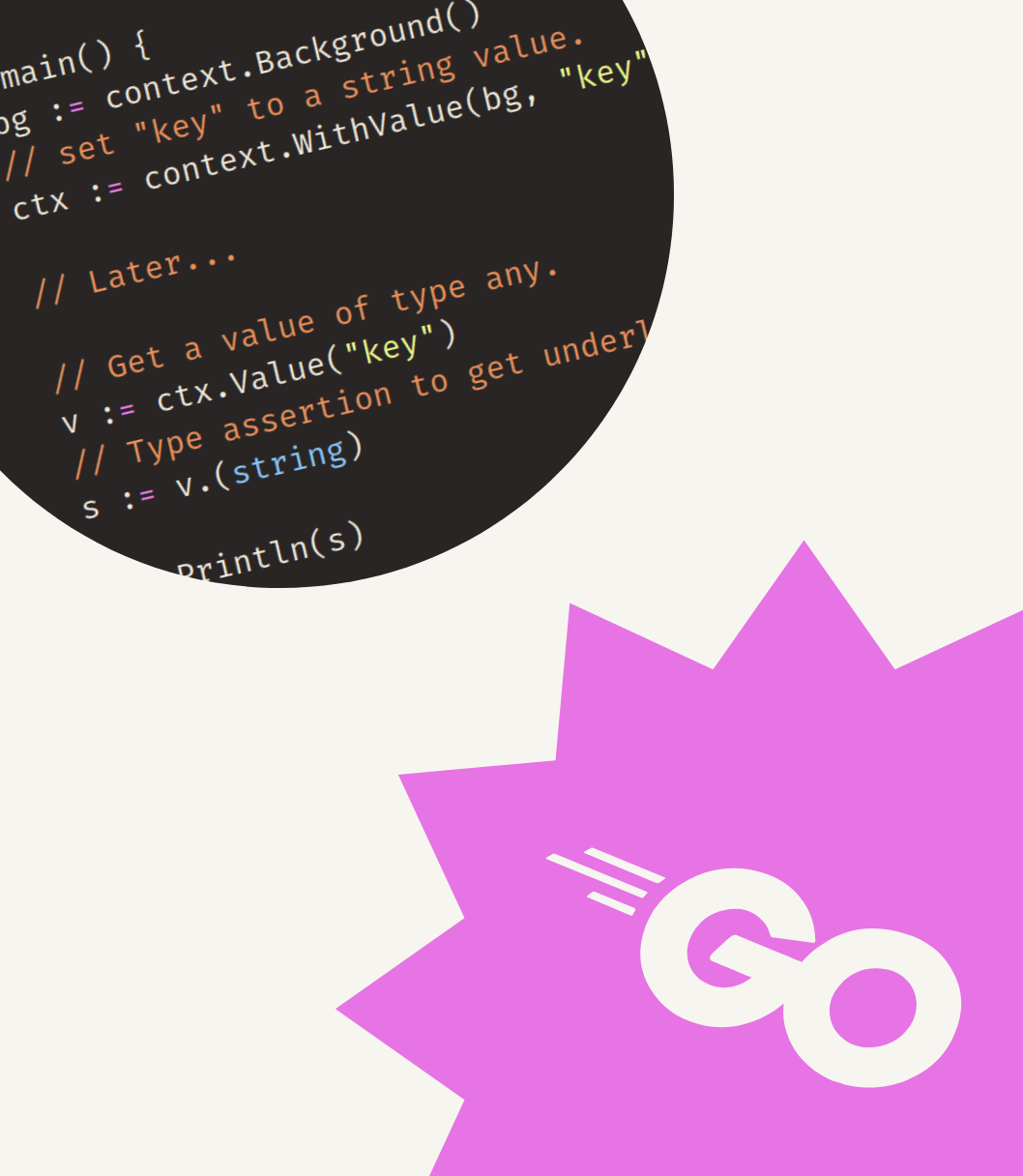
Get my free newsletter every second week
Used by 500+ developers to boost their Go skills.
"I'll share tips, interesting links and new content. You'll also get a brief guide to time for developers for free."
