User preferences, scheduling or legal requirements. There are situations where you will need to convert time.Time
values to UTC.
This snippet will show you how.
Before we dive in, it’s good to know how time.Time
values represent time instants. Learn more here.
Change Location to UTC
Converting a time.Time
to UTC
is done using the UTC()
method on time.Time
.
For example:
package main
import (
"fmt"
"time"
)
func main() {
// t1 is time in "UTC+3" timezone
eastOfUTC := time.FixedZone("UTC+3", 3*60*60)
t1 := time.Date(2024, 01, 22, 13, 37, 0, 0, eastOfUTC)
// t2 represents the same time instant in UTC
t2 := t1.UTC()
fmt.Println(t1.String())
fmt.Println(t2.String())
fmt.Printf("same time instant: %v\n", t1.Equal(t2))
}
In this example, we initially have a time value t1
in the UTC+3
location (a fixed timezone).
We then call t1.UTC()
to create t2
in the UTC
timezone.
t1
and t2
represent the same time instant, but they will have different clock readings. t2
will read 3 hours before t1
.
Run the example to verify this.
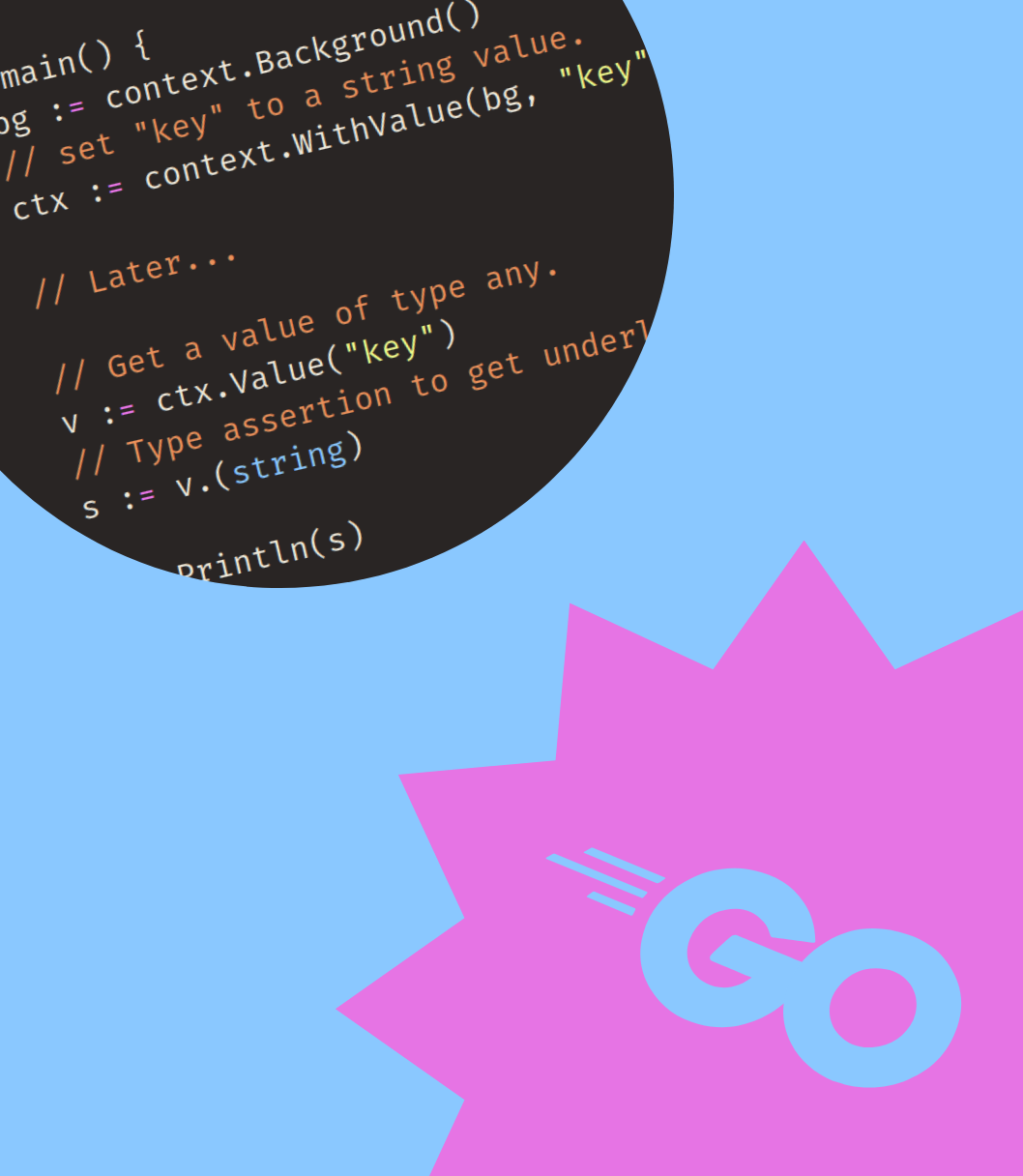
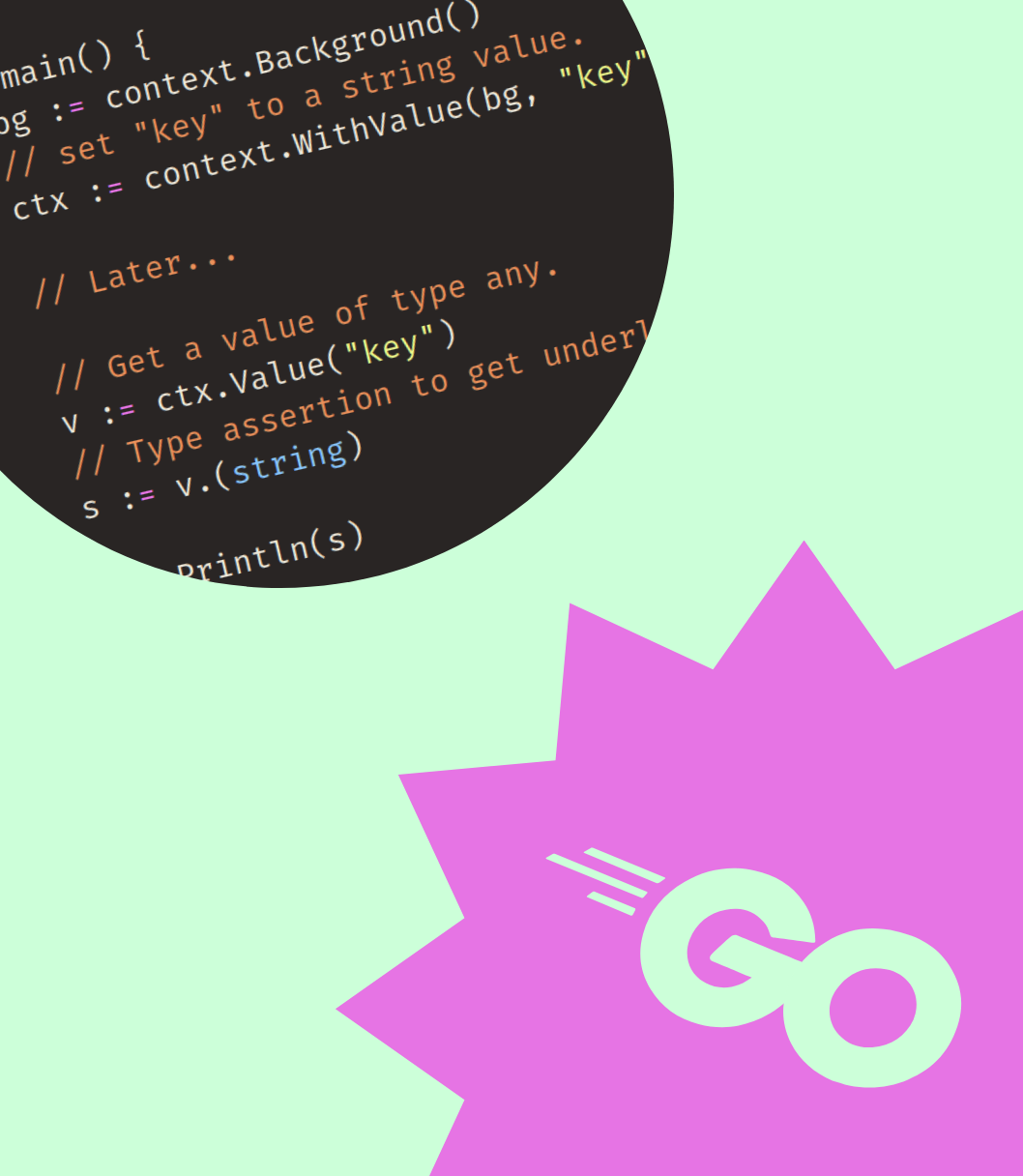
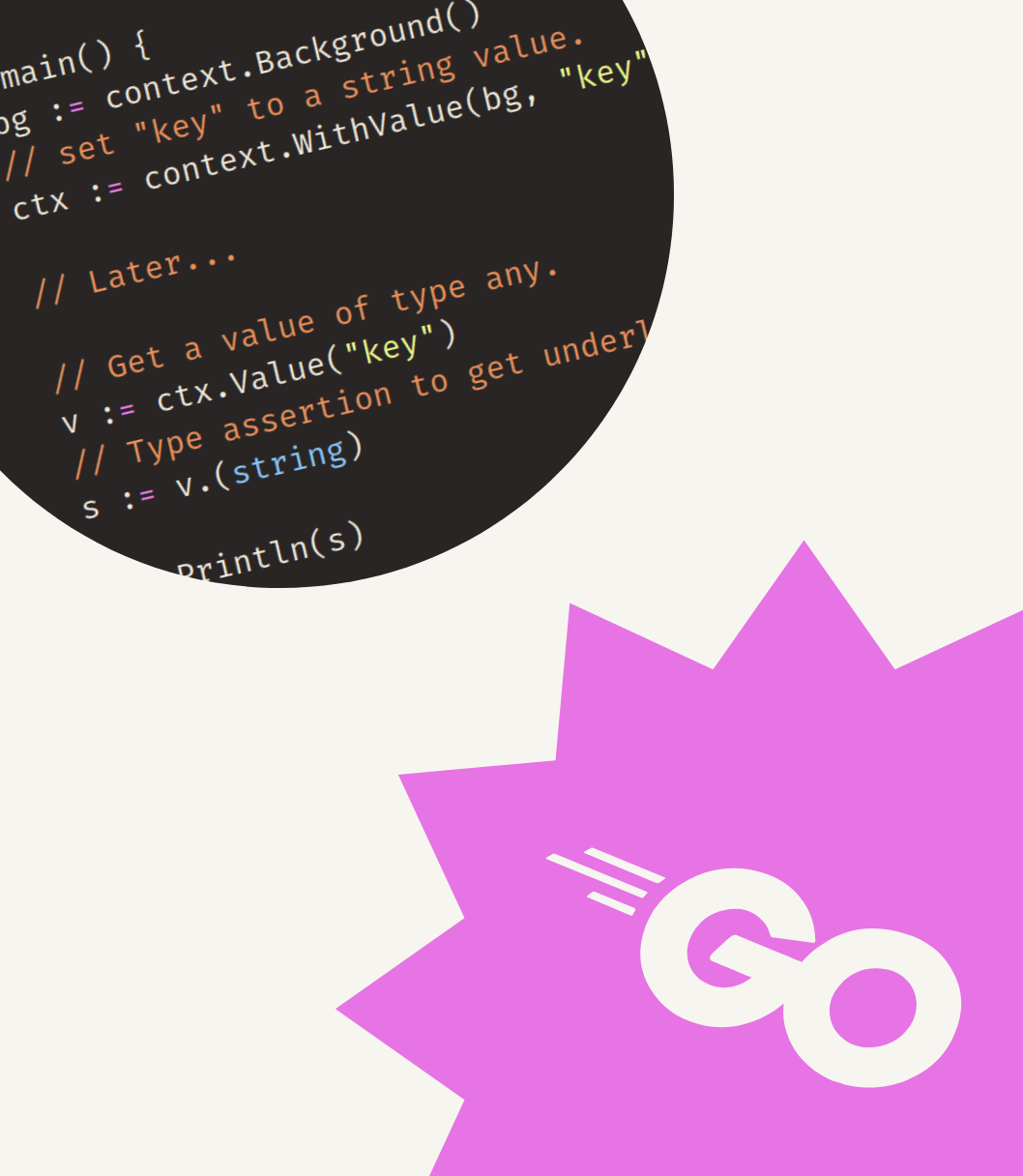
Get my free newsletter every second week
Used by 500+ developers to boost their Go skills.
"I'll share tips, interesting links and new content. You'll also get a brief guide to time for developers for free."
